[고급 C++] 구조체와 구조체포인터
- 컴퓨터공학과 / Programming
- 2020. 10. 27.
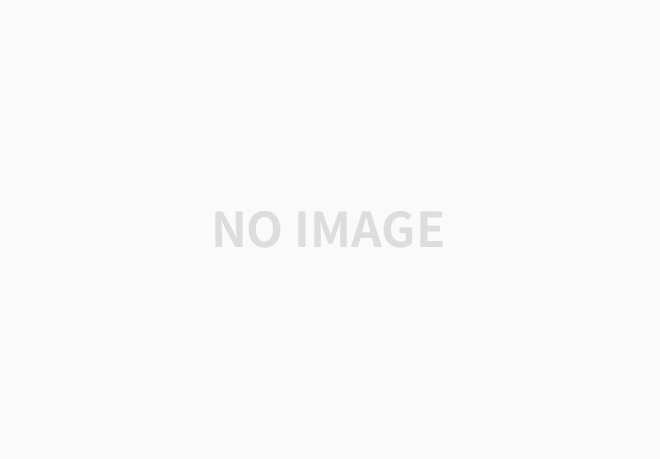
구조체와 구조체포인터 |
구조체
구조체 정의
- 서로 다른 타입의 멤버라고 하는 값들의 통합 자료형
- 사용자 정의 타입
* cf ) 배열 : 같은 타입의 데이터 모임
구조체 선언
#include <stdio.h>
// 변수 아니고 멤버
struct SCORE {
char name[20];
int kor;
int math;
int eng;
float avg;
};
int main()
{
// 구조체 변수
struct SCORE st1 = {"홍길동", 50, 78, 95};
printf("%d, %d \n", sizeof(st1), sizeof(struct SCORE));
printf("&st1 : %p, %p\n", &st1, st1.name);
st1.avg=(st1.kor+st1.math+st1.eng) / (float)3;
printf("%s, %d, %d, %d, %.2f \n", st1.name, st1.kor, st1.math, st1.eng, st1.avg);
return 0;
}
- 선언과 정의를 따로 할 수 있으며, 선언과 정의를 동시에 할 수도 있음
struct SCORE {
char name[20];
int kor;
int math;
int eng;
float avg;
} SCO;
typedef와 구조체 변수
- 재정의된 자료형
typedef struct SCORE {
char name[20];
int kor;
int math;
int eng;
float avg;
} SCO;
// 구조체 변수
struct SCORE st1 = {"홍길동", 50, 78, 95};
SCO tmp;
구조체 메모리 할당
- 구조체의 멤버변수는 주어진 순서대로 메모리에 할당되나
- 빈공간을 포함할 수 있으므로 효율적인 주소 사용을 위한 경계정렬이 필요함
#include <stdio.h>
int main()
{
struct A {
char x; // 1
long int y; // 4
char z; // 1
} tmp1;
struct B {
long int y; // 4
char x; // 1
char z; // 1
} tmp2;
// 멤버 순서만 다른 구조체 크기 확인
printf("tmp1 sizeof : %d \n", sizeof(tmp1));
printf("tmp2 sizeof : %d \n", sizeof(tmp2));
return 0;
}
tmp1 sizeof: 12 |
* tmp1 구조체의 사이즈가 6(=1+4+1)이 아닌 12인 이유
메모리 할당은 4배수(x86) 혹은 8배수(x64)로 메모리를 읽기 때문에 char ☞ 1 (+ 3), long int ☞ 4, char ☞ 1 (+3) 로 계산되어 12Byte 만큼 차지함
- #pragma pack(push, 1) : pop할때까지 현재 설정을 push해서 백업한 뒤 정렬방식 1로 설정
- #pragma pack(pop) : 백업한 것을 현재의 설정으로 되돌림. 정렬 방식 복구
#include <stdio.h>
#pragma pack 1 // 구조체 정렬 방식을 1로함
int main()
{
struct A {
char x;
long int y;
char z;
} tmp;
// 구조체 크기 확인
printf("tmp sizeof : %d \n", sizeof(tmp));
return 0;
}
tmp sizeof : 6 |
구조체 포인터
정의
- 포인터를 통하여 구조체 변수에 접근하는 개념
선언
- 구조체 포인터 변수도 일반 포인터 변수와 동일하게 변수명 앞에 ‘*’를 붙여 선언
- 포인터를 통해 구조체변수에 접근 가능
연산자
- -> : 구조체 포인터 연산자(ptr)
* cf) . : 구조체 멤버 연산자 (st1)
예제
1 #include <stdio.h> 2 3 typedef struct SCORE { 5 char name[20]; // 20 6 int kor; // 4 7 int math; // 4 8 int eng; // 4 9 float avg; // 4 10 } SCO; 11 12 int main() 13 { 14 // 구조체 변수 15 struct SCORE st1 = {"홍길동", 50, 78, 95}; 16 SCO tmp; 17 18 // 구조체 포인트 변수 - 4byte 할당 19 struct SCORE *ptr; 20 21 printf("%d, %d \n", sizeof(st1), sizeof(struct SCORE)); 22 printf("&st1 : %p, %p\n", &st1, st1.name); // 시작 주소 23 25 printf("%s, %d, %d, %d, %.2f \n", st1.name, st1.kor, st1.math, st1.eng, st1.avg); 27 28 tmp = st1; 29 printf("%s, %d, %d, %d, %.2f \n", tmp.name, tmp.kor, tmp.math, tmp.eng, tmp.avg); 31 32 ptr = &st1; 33 printf("%s, %d, %d, %d, %.2f \n", ptr->name, ptr->kor, ptr->math, ptr->eng, ptr->avg); 36 return 0; 37 } |
36, 36 |
- (*ptr).kor == ptr->kor
- 힙은 포인터 접근만 허용함
* 연산자 우선순위
괄호(()) → 배열첨자([]) → 구조체연산(., ->) → 단항연산자(-,!,~,++,--,(type),&,*)
더 많은 시리즈 보기
[고급 C++]C컴파일러와 C라이브러리(공유/정적/동적라이브러리)
[고급 C++]포인터 1편(프로세스/포인터변수/포인터연산/NULL포인터)
궁금한 사항은 댓글로 남겨주세요💃💨💫
좋아요와 구독(로그인X)은 힘이 됩니다 🙈🙉
'컴퓨터공학과 > Programming' 카테고리의 다른 글
[고급 C++] 비트연산자 (0) | 2020.11.02 |
---|---|
[고급 C++] 공용체와 구조체 비트필드 + 바이트 오더링 / 활용방안 (1) | 2020.11.02 |
[고급 C++] 가변인자 / CERT C (feat. 안전 코딩하기) (1) | 2020.10.26 |
[고급 C++]포인터 4편(다중 포인터/함수 포인터/void형 포인터) (1) | 2020.10.23 |
[고급 C++]포인터 3편(배열 포인터/포인터 배열/문자열 상수 포인터) (2) | 2020.10.22 |